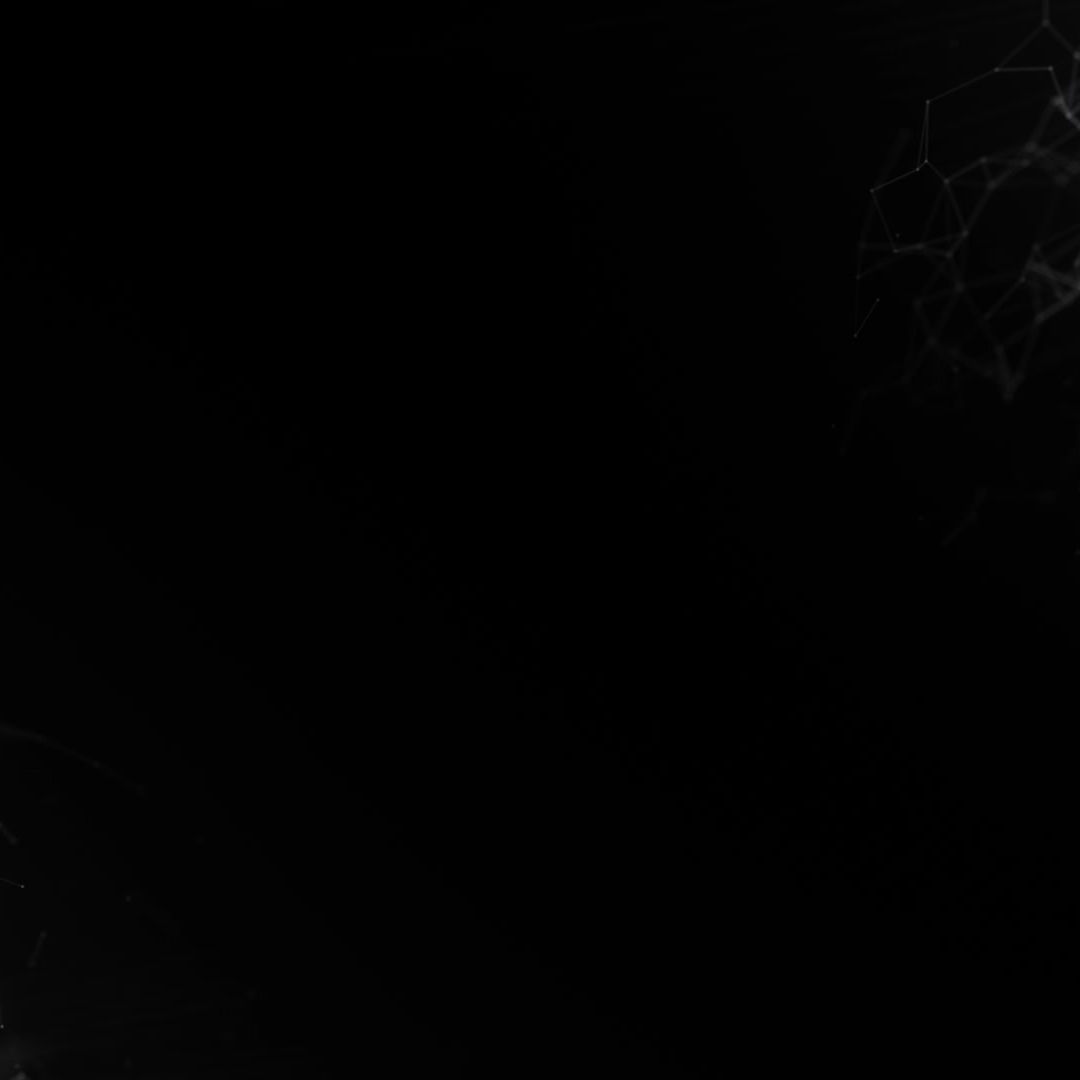
Meowster - LEDS & Audio Code
import time
import board
import neopixel
from digitalio import DigitalInOut, Direction, Pull
from adafruit_led_animation.animation.rainbowsparkle import RainbowSparkle
from adafruit_led_animation.animation.rainbow import Rainbow
from adafruit_led_animation.sequence import AnimationSequence
from adafruit_led_animation.animation.pulse import Pulse
from adafruit_led_animation.animation.blink import Blink
from adafruit_led_animation.color import (
WHITE,
TEAL
)
pixel_pin = board.A1
num_pixels = 100
stopcycle = 0
pixels = neopixel.NeoPixel(pixel_pin, num_pixels, brightness=0.3, auto_write=False)
rainbow_sparkle = RainbowSparkle(pixels, speed=0.1, num_sparkles=15, period=15)
rainbow = Rainbow(pixels, speed=0.1, period=2)
CYAN = (0, 255, 255)
RED = (255, 0, 0)
BLUE = (0, 0, 255)
spreeBlink = Blink(pixels, speed=0.01, color=RED)
R2 = RainbowSparkle(pixels, speed=0.1, num_sparkles=15)
def health_improve(color, wait):
for i in range(num_pixels):
pixels[i] = color
time.sleep(wait)
pixels.show()
time.sleep(0.00001)
def health_bad(color, wait):
for i in range(num_pixels):
pixels[-i] = color
time.sleep(wait)
pixels.show()
time.sleep(0.00001)
def color_chase(color, wait):
for i in range(num_pixels):
pixels[i] = color
time.sleep(wait)
pixels.show()
time.sleep(0.00001)
bowpulse = Pulse(pixels, speed=0.001, color=TEAL, period=3)
bowpulse2 = Pulse(pixels, speed=0.00001, color=TEAL, period=1)
restTeal = Pulse(pixels, speed=0.07, color=TEAL, period=5)
pulse = Pulse(pixels, speed=0.01, color=CYAN, period=3)
health = Pulse(pixels, speed=0.1, color=CYAN, period=3)
haloIntro = Pulse(pixels, speed=0.01, color=WHITE, period=6)
haloIntro2 = Pulse(pixels, speed=0.01, color=WHITE, period=2)
haloIntro3 = Pulse(pixels, speed=0.01, color=RED, period=3)
haloIntro4 = Pulse(pixels, speed=0.01, color=TEAL, period=3)
killingspree = Pulse(pixels, speed=0.01, color=RED, period=2)
def offcolor(wait):
pixels.fill((0, 0, 0))
time.sleep(wait)
pixels.show()
time.sleep(.01)
def shotsFired(wait):
pixels.fill(RED)
time.sleep(wait)
pixels.show()
time.sleep(.01)
def bowchicka(wait):
pixels.fill(CYAN)
time.sleep(wait)
pixels.show()
time.sleep(.01)
switch5 = DigitalInOut(board.D5)
switch5.direction = Direction.INPUT
switch5.pull = Pull.UP
switch6 = DigitalInOut(board.D6)
switch6.direction = Direction.INPUT
switch6.pull = Pull.UP
switch9 = DigitalInOut(board.D9)
switch9.direction = Direction.INPUT
switch9.pull = Pull.UP
switch10 = DigitalInOut(board.D10)
switch10.direction = Direction.INPUT
switch10.pull = Pull.UP
switch11 = DigitalInOut(board.D11)
switch11.direction = Direction.INPUT
switch11.pull = Pull.UP
birthdayAnimations = AnimationSequence(
rainbow,
rainbow_sparkle,
advance_interval=2,
auto_clear=True,
)
bowChicaanimations = AnimationSequence(
bowpulse2,
advance_interval=1,
auto_clear=True,
)
Halointr = AnimationSequence(
haloIntro2, haloIntro4, haloIntro3,
advance_interval=1,
auto_clear=True,
)
halotheme = AnimationSequence(
pulse, pulse, rainbow, rainbow_sparkle,
advance_interval=20,
auto_clear=True,
)
fullHealthUp = AnimationSequence(
health,
advance_interval=1,
auto_clear=True,
)
Restingphase = AnimationSequence(
restTeal,
advance_interval=1000,
auto_clear=True,
)
while True:
Restingphase.animate()
time.sleep(.2)
if not switch5.value:
# birthday party
while True:
birthdayAnimations.animate()
if stopcycle < 30:
time.sleep(.1)
stopcycle = stopcycle+1
else:
stopcycle = 0
offcolor(0)
time.sleep(.05)
break
if not switch6.value:
# bowchikawowow
while True:
if stopcycle == 0:
time.sleep(.2)
stopcycle = stopcycle+1
if stopcycle == 1:
health_improve(CYAN, 0.00001)
time.sleep(.008)
stopcycle = stopcycle+1
if stopcycle > 1 and stopcycle < 12:
rainbow.animate()
time.sleep(.08)
stopcycle = stopcycle+1
if stopcycle >= 12:
stopcycle = 0
offcolor(0)
time.sleep(.05)
break
if not switch9.value:
# killing spree
while True:
if stopcycle == 0:
time.sleep(.35)
stopcycle = stopcycle+1
if stopcycle >= 1 and stopcycle < 4:
time.sleep(.05)
shotsFired(.1)
time.sleep(.1)
offcolor(0)
stopcycle = stopcycle+1
if stopcycle == 3:
time.sleep(1)
stopcycle = stopcycle+1
if stopcycle >= 4 and stopcycle < 7:
time.sleep(.05)
shotsFired(.1)
time.sleep(.1)
offcolor(0)
stopcycle = stopcycle+1
if stopcycle == 7:
time.sleep(.5)
stopcycle = stopcycle+1
if stopcycle >= 8 and stopcycle < 12:
time.sleep(.05)
shotsFired(.1)
time.sleep(.1)
offcolor(0)
stopcycle = stopcycle+1
if stopcycle == 12:
time.sleep(.2)
stopcycle = stopcycle+1
if stopcycle >= 13 and stopcycle < 40:
time.sleep(.09)
spreeBlink.animate()
stopcycle = stopcycle+1
if stopcycle >= 39 and stopcycle < 50:
time.sleep(.08)
spreeBlink.animate()
stopcycle = stopcycle+1
if stopcycle >= 50 and stopcycle < 90:
killingspree.animate()
stopcycle = stopcycle+1
time.sleep(.05)
if stopcycle >= 90 and stopcycle < 92:
health_improve(CYAN, 0.00001)
health_bad(RED, 0.000001)
time.sleep(.05)
stopcycle = stopcycle+1
if stopcycle >= 92 and stopcycle < 120:
time.sleep(.07)
rainbow.animate()
stopcycle = stopcycle+1
if stopcycle >= 120:
stopcycle = 0
offcolor(0)
time.sleep(.05)
break
if not switch10.value:
# Halo Intro Theme Song
time.sleep(3)
while True:
# 602 seconds long
if stopcycle < 173:
haloIntro.animate()
time.sleep(.1)
stopcycle = stopcycle+1
if stopcycle > 105 and stopcycle < 115:
spreeBlink.animate()
time.sleep(.1)
stopcycle = stopcycle+1
if stopcycle > 172 and stopcycle < 297:
haloIntro2.animate()
time.sleep(.08)
stopcycle = stopcycle+1
if stopcycle > 296 and stopcycle < 310:
rainbow.animate()
time.sleep(.1)
stopcycle = stopcycle+1
if stopcycle > 309:
stopcycle = 0
offcolor(0)
time.sleep(.05)
break
if not switch11.value:
# Energy Shield Depleted
time.sleep(2.5)
while True:
if stopcycle < 10:
spreeBlink.animate()
time.sleep(.08)
stopcycle = stopcycle+1
if stopcycle > 9 and stopcycle < 20:
stopcycle = stopcycle+1
bowChicaanimations.animate()
time.sleep(.05)
spreeBlink.animate()
time.sleep(.05)
spreeBlink.animate()
time.sleep(.04)
bowChicaanimations.animate()
time.sleep(.05)
spreeBlink.animate()
time.sleep(.05)
spreeBlink.animate()
time.sleep(.04)
stopcycle = stopcycle+1
if stopcycle == 20:
offcolor(.2)
stopcycle = stopcycle+1
if stopcycle > 20:
health_improve(CYAN, 0.01)
time.sleep(.1)
stopcycle = stopcycle+1
if stopcycle == 29:
time.sleep(1)
if stopcycle > 30:
health_bad((0, 0, 0), 0.01)
time.sleep(.1)
stopcycle = stopcycle+1
if stopcycle == 31:
time.sleep(1)
if stopcycle > 31:
stopcycle = 0
offcolor(0)
time.sleep(.05)
break